Drag and Drop File Upload Using Dropzone JS and PHP
Mar 12, 2022
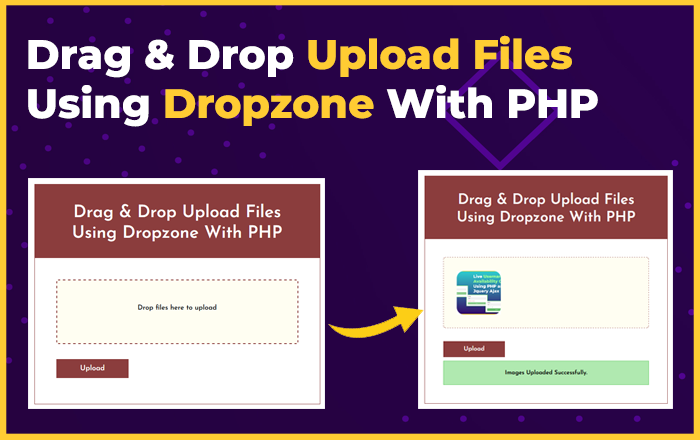
index.php
<?php
$conn = mysqli_connect("localhost", "root", "", "dropzone");
if (!$conn) {
die("Connection failed: " . mysqli_connect_error());
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Drag & Drop Using Dropzone With PHP</title>
<link rel="stylesheet" href="css/style.css">
<link rel="stylesheet" href="css/dropzone.css"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
</head>
<body>
<div id="main">
<div class="container">
<div class="row">
<div class="col-md-7 div-center col-11">
<div id="header">
<h1>Drag & Drop Upload Files<br> Using Dropzone With PHP</h1>
</div>
<div id="content">
<form class="dropzone" id="file_upload"></form>
<button id="upload_btn">Upload</button>
</div>
</div>
</div>
</div>
</div>
<?php
$swl = "select * from images WHERE ID='16'";
$d = mysqli_query($conn, $swl);
while ($row = mysqli_fetch_assoc($d)) {
$img = $row['images'];
$myArray = explode(',', $img);
$string = preg_replace('/\s+/', '', $myArray);
?>
<?php } ?>
<script type="text/javascript" src="js/jquery.js"></script>
<script type="text/javascript" src="js/dropzone.js"></script>
<script>
Dropzone.autoDiscover = false;
var myDropzone = new Dropzone("#file_upload", {
url: "upload.php",
parallelUploads: 3,
uploadMultiple: true,
acceptedFiles: '.png,.jpg,.jpeg',
autoProcessQueue: false,
success: function(file, response) {
if (response == 'true') {
$('#content .message').hide();
$('#content').append('<div class="message success">Images Uploaded Successfully.</div>');
} else {
$('#content').append('<div class="message error">Images Can\'t Uploaded.</div>');
}
}
});
$('#upload_btn').click(function() {
myDropzone.processQueue();
});
</script>
</body>
</html>
upload.php
<?php
$conn = mysqli_connect("localhost", "root", "","dropzone");
if (!$conn) {
die("Connection failed: " . mysqli_connect_error());
}
if($_FILES['file']['name'] != ''){
$file_names = '';
$total = count($_FILES['file']['name']);
for($i=0; $i<$total; $i++){
$filename = $_FILES['file']['name'][$i];
$extension = pathinfo($filename,PATHINFO_EXTENSION);
$valid_extensions = array("png","jpg","jpeg");
if(in_array($extension, $valid_extensions)){
$new_name = rand() . ".". $extension;
$path = "images/" . $new_name;
move_uploaded_file($_FILES['file']['tmp_name'][$i], $path);
$file_names .= $new_name . " , ";
}else{
echo 'false';
}
}
$sql = "INSERT INTO images(images) VALUES('{$file_names}')";
if(mysqli_query($conn,$sql)){
echo 'true';
}else{
echo 'false';
}
}
style.css
@import url('https://fonts.googleapis.com/css2?family=Josefin+Sans:wght@600&display=swap');
body {
font-family: 'Josefin Sans', sans-serif !important;
padding: 0;
margin: 0;
}
.center {
text-align: center;
}
.div-center {
margin: 0 auto;
border: 1px solid #8b3d3d;
padding: 0 !important;
}
#main {
margin: 0 auto;
margin-top: 25px;
}
#header {
background: #8b3d3d !important;
color: #fff;
height: auto;
padding: 25px;
}
h1 {
line-height: 51px;
font-size: 38px;
text-align: center;
margin: 0px;
padding-top: 17px;
}
#content {
min-height: 300px;
padding: 50px;
}
form {
margin: 0 0 10px;
}
#upload_btn {
background: #8b3d3d;
border: 1px solid #8b3d3d;
outline: 0;
padding: 8px 15px;
margin: 0 0 10px;
margin-top: 0px;
color: #fff;
font-size: 17px;
border-radius: 0;
cursor: pointer;
width: 30%;
margin-top: 25px;
}
#upload_btn:hover {
background: transparent;
color: #8b3d3d;
transition: all 0.5s ease;
}
.message {
color: #000;
background-color: #e7e7e7;
padding: 20px;
font-size: 16px;
font-weight: 600;
text-align: center;
}
.message.success {
color: #000;
background-color: #b0e9b0;
border: 1px solid #6dce6d;
}
.message.error {
color: #fff;
background-color: #f37d7d;
border: 1px solid #e91919;
}