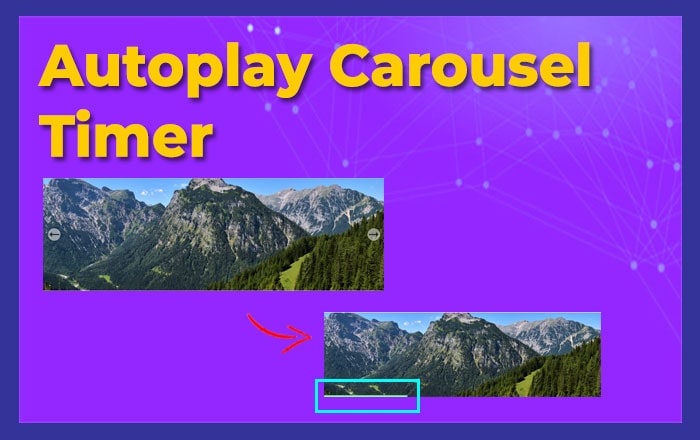
Folder Structure
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="css/jquery.carousel-line-arrow.css" />
<link rel="stylesheet" href="css/style.css" />
<link href="https://www.jqueryscript.net/css/jquerysctipttop.css" rel="stylesheet" type="text/css" />
<title>Carousel With Navigation Arrows And Autoplay Timer Example</title>
</head>
<body>
<div class="wrapper">
<div class="your-slider">
<div class="your-slider-item">
<img src="https://source.unsplash.com/1600x900/?nature" alt="" />
</div>
<div class="your-slider-item">
<img src="https://source.unsplash.com/1600x900/?mountain" alt="" />
</div>
<div class="your-slider-item">
<img src="https://source.unsplash.com/1600x900/?computer" alt="" />
</div>
<div class="your-slider-item">
<img src="https://source.unsplash.com/1600x900/?hacker" alt="" />
</div>
</div>
</div>
<script src="https://code.jquery.com/jquery-3.4.1.min.js" integrity="sha256-CSXorXvZcTkaix6Yvo6HppcZGetbYMGWSFlBw8HfCJo=" crossorigin="anonymous"></script>
<script src="js/jquery.carousel-line-arrow.js"></script>
<script src="js/main.js"></script>
</body>
</html>
style.css
* {
margin: 0;
padding: 0;
}
body .wrapper {
max-width: 100%;
}
body .wrapper .your-slider {
width: 100%;
}
.carousel-wrapper-middle {
height: 450px;
}
jquery.carousel-line-arrow.css
.carouselLineArrow-line {
position: absolute;
left: 0;
width: 100%;
}
.carouselLineArrow-line .carouselLineArrow-innerline {
height: 100%;
width: 0%;
}
.carouselLineArrow-arrow {
position: absolute;
width: 50px;
height: 50px;
border-radius: 50%;
background-color: rgba(255, 255, 255, 0.5);
top: 50%;
-webkit-transform: translateY(-50%);
transform: translateY(-50%);
-webkit-transition: .3s;
transition: .3s;
cursor: pointer;
-webkit-box-shadow: 0 0 10px 0px rgba(0, 0, 0, 0.5);
box-shadow: 0 0 10px 0px rgba(0, 0, 0, 0.5);
}
.carouselLineArrow-arrow:hover {
background-color: white;
}
.carouselLineArrow-arrow.carouselLineArrow-arrow-left {
left: -50px;
}
.carouselLineArrow-arrow.carouselLineArrow-arrow-left:hover .carouselLineArrow-arrow-inner {
-webkit-transform: rotate(-360deg);
transform: rotate(-360deg);
}
.carouselLineArrow-arrow.carouselLineArrow-arrow-left .carouselLineArrow-arrow-inner {
height: 100%;
width: 100%;
-webkit-transition: .3s;
transition: .3s;
}
.carouselLineArrow-arrow.carouselLineArrow-arrow-left .carouselLineArrow-arrow-inner:before {
position: absolute;
content: '';
background-color: #222;
height: 4px;
width: 30px;
top: 50%;
right: 5px;
-webkit-transform: translate(0%, -50%);
transform: translate(0%, -50%);
}
.carouselLineArrow-arrow.carouselLineArrow-arrow-left .carouselLineArrow-arrow-inner:after {
position: absolute;
content: '';
height: 0px;
width: 0px;
border: 10px solid transparent;
border-right: 10px solid #222;
top: 50%;
right: 34px;
-webkit-transform: translate(0, -50%);
transform: translate(0, -50%);
}
.carouselLineArrow-arrow.carouselLineArrow-arrow-right {
right: -50px;
}
.carouselLineArrow-arrow.carouselLineArrow-arrow-right:hover .carouselLineArrow-arrow-inner {
-webkit-transform: rotate(360deg);
transform: rotate(360deg);
}
.carouselLineArrow-arrow.carouselLineArrow-arrow-right .carouselLineArrow-arrow-inner {
height: 100%;
width: 100%;
-webkit-transition: .3s;
transition: .3s;
}
.carouselLineArrow-arrow.carouselLineArrow-arrow-right .carouselLineArrow-arrow-inner:before {
position: absolute;
content: '';
background-color: #222;
height: 4px;
width: 30px;
top: 50%;
left: 5px;
-webkit-transform: translate(0%, -50%);
transform: translate(0%, -50%);
}
.carouselLineArrow-arrow.carouselLineArrow-arrow-right .carouselLineArrow-arrow-inner:after {
position: absolute;
content: '';
height: 0px;
width: 0px;
border: 10px solid transparent;
border-left: 10px solid #222;
top: 50%;
left: 34px;
-webkit-transform: translate(0, -50%);
transform: translate(0, -50%);
}
.carousel-wrapper-middle:hover .carouselLineArrow-arrow.carouselLineArrow-arrow-left {
left: 20px;
}
.carousel-wrapper-middle:hover .carouselLineArrow-arrow.carouselLineArrow-arrow-right {
right: 20px;
}
main.js
$(function ()
{
$('.your-slider').carouselLineArrow(
{
lineDur: 6000, //duration of line-time animation (ms), default is 5000
slideDur: 600, //duration of toggle slide animation (ms), default is 500
heightIsProportional: true, // height of slider is proportional to the width when resized, defaultl is true
linePosition: 'bottom', // position of line-time: 'bottom' or 'top', default is 'bottom'
lineHeight: '10px', // height of line-time (px, em, rem, %), default is '5px';
lineColor: '#7fff95' // color of line-time, default is 'red'
});
})
jquery.carousel-line-arrow.js
(function ($)
{
jQuery.fn.carouselLineArrow = function (options)
{
options = $.extend(
{
lineDur: 5000, //duration of line-time animation (ms), default is 5000
slideDur: 500, //duration of toggle slide animation (ms), default is 500
heightIsProportional: true, // height of slider is proportional to the width when resized, defaultl is true
linePosition: "bottom", // position of line-time: 'bottom' or 'top', default is 'bottom'
lineHeight: "5px", // height of line-time (px, em, rem, %), default is '5px';
lineColor: "red", // color of line-time, default is 'red'
}, options);
let make = function ()
{
$(this).css("overflow", "hidden");
let $this = $(this);
let items = $(this).children();
let imgItem = items.first().children();
let imgsItem = items.children();
let imgWidth = imgItem.width();
let imgHeight = imgItem.height();
let proportial = imgHeight / imgWidth;
$(this).wrap("<div class='carousel-wrapper-middle'></div>");
$(".carousel-wrapper-middle").wrap("<div class='carousel-wrapper-outer'></div>");
$(".carousel-wrapper-outer").css("width", "100%");
$(".carousel-wrapper-middle").css("width", "100%");
if (options.heightIsProportional)
{
$(".carousel-wrapper-middle").css("padding-bottom", proportial * 100 + "%");
}
else
{
wrapperWidth = $(".carousel-wrapper-outer").width();
$(".carousel-wrapper-middle").css("padding-bottom",
(imgHeight * wrapperWidth) / imgWidth);
}
$(".carousel-wrapper-middle").css("position", "relative");
$(this).css("position", "absolute");
$(this).css("width", "100%");
$(this).css("height", "100%");
$(this).css("top", "0%");
$(this).css("left", "0%");
items.css("position", "absolute");
items.css("width", "100%");
items.css("height", "100%");
items.css("top", "0%");
items.css("left", "0%");
//imgsItem.css('max-width', '100%');
imgsItem.css("width", "100%");
imgsItem.css("height", "100%");
imgsItem.css("object-fit", "cover");
items.css("display", "none");
items.eq(0).css("display", "block");
$(this).append('<div class="carouselLineArrow-line"><div class="carouselLineArrow-innerline"></div></div>');
let line = $(this).children(".carouselLineArrow-line");
line.css(options.linePosition, "0");
line.css("height", options.lineHeight);
let innerLine = line.children(".carouselLineArrow-innerline");
innerLine.css("background-color", options.lineColor);
$(this).append('<div class="carouselLineArrow-arrow carouselLineArrow-arrow-right"><div class="carouselLineArrow-arrow-inner"></div></div>');
$(this).append('<div class="carouselLineArrow-arrow carouselLineArrow-arrow-left"><div class="carouselLineArrow-arrow-inner"></div></div>');
let arrowLeft = $this.children(".carouselLineArrow-arrow-left");
let arrowRight = $this.children(".carouselLineArrow-arrow-right");
let iLast = items.length - 1;
let iCurr = 0;
let iNext;
let iPrev;
let sliderHover = false;
let lineIsAnim = false;
let slideIsAnim = false;
function nextI(i)
{
return i < iLast ? i + 1 : 0;
}
function prevI(i)
{
return i > 0 ? i - 1 : iLast;
}
function afterSlideAnimateLeft()
{
slideIsAnim = false;
items.each(function (index)
{
if (index !== iCurr)
{
items.eq(index).css("display", "none");
}
});
lineIsAnim = true;
//console.log(`lineIsAnim = ${lineIsAnim}`);
if (!sliderHover)
{
innerLine.animate(
{
width: "100%",
}, options.lineDur, afterLineAnimateLeft);
}
}
function afterLineAnimateLeft()
{
lineIsAnim = false;
innerLine.css("width", "0");
iNext = nextI(iCurr);
items.eq(iCurr).css("display", "block");
items.eq(iNext).css("display", "block");
items.eq(iCurr).css("left", "0%");
items.eq(iNext).css("left", "99%");
slideIsAnim = true;
items.eq(iCurr).animate(
{
left: "-99%",
}, options.slideDur, afterSlideAnimateLeft);
items.eq(iNext).animate(
{
left: "0%",
}, options.slideDur);
if (iCurr < iLast)
{
iCurr++;
}
else
{
iCurr = 0;
}
}
function afterLineAnimateRight()
{
lineIsAnim = false;
innerLine.css("width", "0");
iPrev = prevI(iCurr);
items.eq(iCurr).css("display", "block");
items.eq(iPrev).css("display", "block");
items.eq(iCurr).css("left", "0%");
items.eq(iPrev).css("left", "-99%");
slideIsAnim = true;
items.eq(iCurr).animate(
{
left: "99%",
}, options.slideDur, afterSlideAnimateLeft);
items.eq(iPrev).animate(
{
left: "0%",
}, options.slideDur);
if (iCurr > 0)
{
iCurr--;
}
else
{
iCurr = iLast;
}
}
lineIsAnim = true;
if (!sliderHover)
{
innerLine.animate(
{
width: "100%",
}, options.lineDur, afterLineAnimateLeft);
}
$(this).on("mouseenter", function ()
{
sliderHover = true;
if (lineIsAnim)
{
innerLine.stop();
innerLine.css("width", "0%");
lineIsAnim = false;
}
});
$(this).on("mouseleave", function ()
{
sliderHover = false;
if (!slideIsAnim)
{
lineIsAnim = true;
if (!sliderHover)
{
innerLine.animate(
{
width: "100%",
}, options.lineDur, afterLineAnimateLeft);
}
}
});
arrowLeft.on("click", function ()
{
if (!slideIsAnim)
{
afterLineAnimateLeft();
}
});
arrowRight.on("click", function ()
{
if (!slideIsAnim)
{
afterLineAnimateRight();
}
});
};
return this.each(make);
};
})(jQuery);
3.91 KB