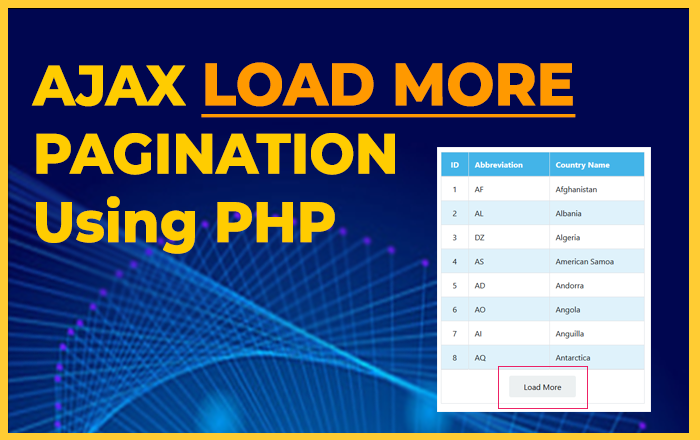
index.php
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>PHP Ajax Load More Pagination</title>
<link rel="stylesheet" href="css/style.css">
<!-- Latest compiled and minified CSS -->
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
<!-- jQuery library -->
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<!-- Latest compiled JavaScript --> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js"></script>
</head>
<body>
<div id="main" class="mt-5">
<div class="container">
<div class="row">
<div class="col-md-5 center">
<div id="table-data">
<div class="table-responsive">
<table id="loadData" class="table table-bordered">
<tr>
<th class="text-center">ID</th>
<th>Abbreviation</th>
<th>Country Name</th>
</tr>
</table>
</div>
</div>
</div>
</div>
</div>
</div>
<script>
$(document).ready(function() {
// Load Data from Database with Ajax
function loadTable(page) {
$.ajax({
url: "ajax-pagination.php",
type: "POST",
data: {
page_no: page
},
success: function(data) {
if (data) {
$("#pagination").remove();
$("#loadData").append(data);
} else {
$("#ajaxbtn").hide();
$("#ajaxbtn").prop("disabled", true);
}
}
});
}
loadTable();
// Add Click Event on ajaxbtn
$(document).on("click", "#ajaxbtn", function() {
$("#ajaxbtn").html("Loading...");
var pid = $(this).data("id");
loadTable(pid);
});
});
</script>
</body>
</html>
ajax-pagination.php
<?php
$conn = mysqli_connect("localhost","root","","pagination") or die("Connection failed");
$limit = 8;
if(isset($_POST['page_no'])){
$page = $_POST['page_no'];
}else{
$page = 0;
}
$sql = "SELECT * FROM countries LIMIT {$page},$limit";
$query = mysqli_query($conn,$sql) or die("Query Unsuccessful.");
if(mysqli_num_rows($query) > 0){
$output = "";
$output .= "<tbody>";
while($row = mysqli_fetch_assoc($query)) {
$last_id = $row["id"];
$output .= "<tr><td align='center'>{$row["id"]}</td><td>{$row["sortname"]}</td><td>{$row["name"]} </td></tr>";
}
$output .= "</tbody>
<tbody id='pagination'>
<tr>
<td colspan='3'>
<button id='ajaxbtn' data-id='{$last_id}'>Load More</button>
</td>
</tr>
</tbody>";
echo $output;
}else{
echo "";
}
mysqli_close($conn);
?>
style.css
body {
font-family: arial;
background: #b2bec3;
padding: 0;
margin: 0;
}
h1 {
text-align: center;
margin: 0;
}
.center {
margin: 0 auto;
}
#table-data {
padding: 15px;
min-height: 500px;
}
#table-data th {
background: #43b4e8;
color: #fff;
}
#table-data tbody tr:nth-child(even) {
background: #ecf0f1;
}
#pagination {
text-align: center;
}
#pagination tr {
background: #fff;
}
#ajaxbtn {
background: #ecf0f1;
color: #131313;
border: 0;
outline: 0;
font-size: 16px;
padding: 10px 30px;
border-radius: 4px;
cursor: pointer;
}
#ajaxbtn:disabled {
background: #7f8c8d;
color: #2c3e50;
cursor: default;
}
#table-data tbody tr:nth-child(2n) {
background: #43b4e82b;
}
5.66 KB